Node.js
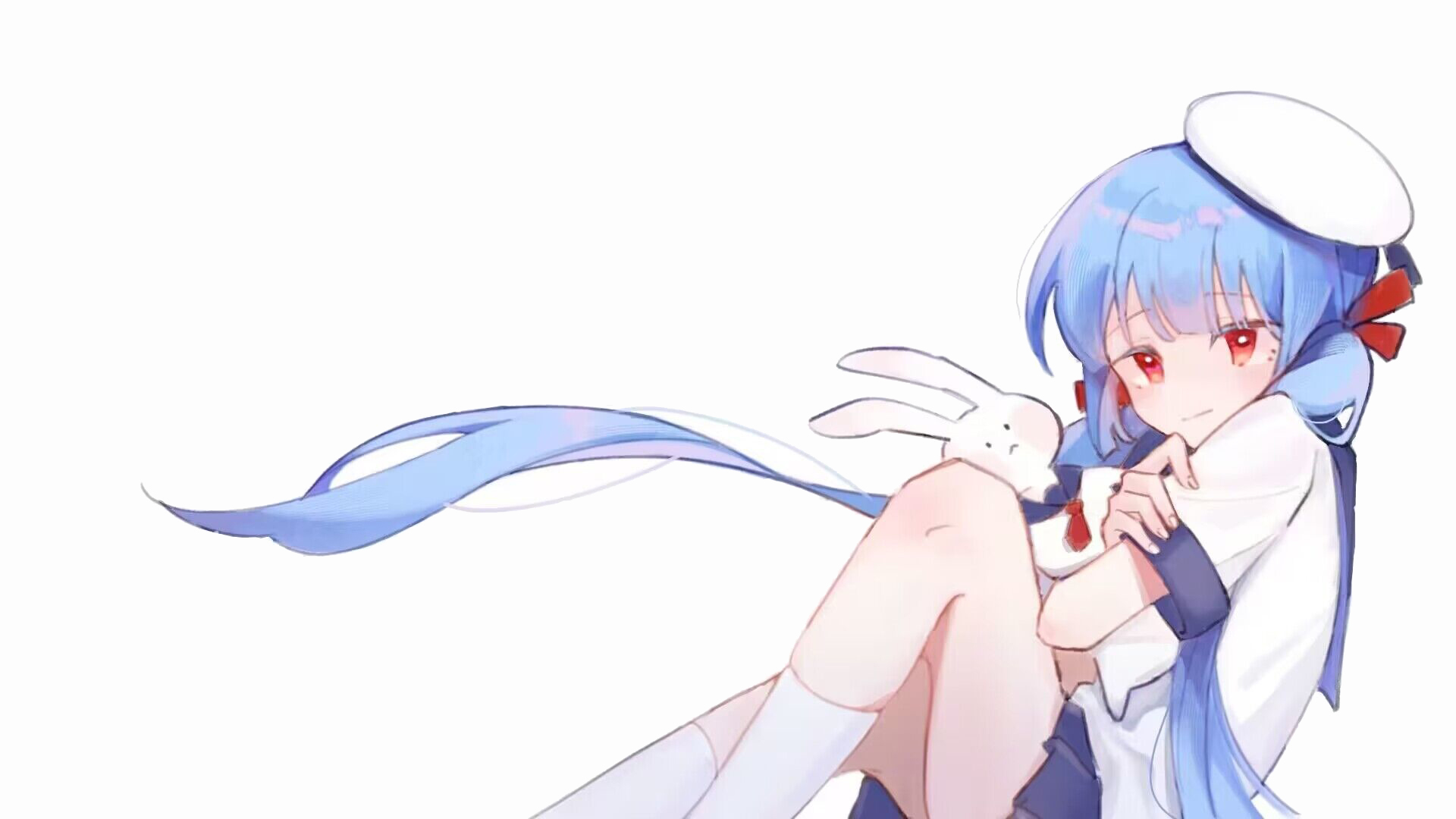
Milkio can freely switch JavaScript runtimes and can run in Node.js.
Node.js is currently the most widely used JavaScript runtime, accompanying JavaScript throughout its growth.
Installing Node.js
You can go to this link to install Node.js, or use nvm or nvm-windows to easily switch between versions.
After installation, by running the following command, if the version number is displayed successfully, it means your Node.js has been installed successfully.
node --version && npm --version
Using Node.js
Node.js does not have built-in support for running TypeScript files directly, we can achieve this by installing vite-node.
npm install --save-dev vite-node
Edit your /package.json
file, replace the commands using Bun to run with the commands using vite-node.
{ "scripts": { "start": "vite-node ./run-serve.ts", "dev": "vite-node ./run-serve.ts", "api-test": "vite-node ./run-api-test.ts", "command": "vite-node ./run-command.ts", ... },}
Since using the built-in HTTP server in Node.js can be complex, we recommend using Hattip to simplify this process.
npm install --save @hattip/adapter-node # Install Hattip
Edit your /run-serve.ts
file, change the code for starting the HTTP server in default mode to use Hattip.
import { defineHttpHandler, envToNumber } from "milkio";import { env } from "node:process";import { milkio } from "./milkio";import { createServer } from "@hattip/adapter-node"
async function serve() { const httpHandler = defineHttpHandler(await milkio); // if you are using Bun Bun.serve({ port: envToNumber(env.PORT, 9000), fetch(request) { return httpHandler({ request }); }, }); // if you are using Hattip createServer(httpHandler).listen(envToNumber(env.PORT, 9000), "localhost", () => { console.log(`Server listening on http://localhost:${envToNumber(env.PORT, 9000)}`) })}
serve();
Deployment
We don’t need to install bundling tools like Rollup or ESBuild because Bun itself supports bundling code for Node.js and is faster than ESBuild.
Modify our /package.json
file, replace the build
command in the scripts
section with:
bun build ./run-serve.ts --splitting --sourcemap=inline --outdir=./dist --target=node --format=esm --minify
When we run npm run build
, our code will be bundled into the dist
directory. Copy the directory to your server, and you can run it directly with Node.js:
cd ./dist && node ./run-serve.js
If it doesn’t run, try renaming the file to run-serve.mjs
.
Details
By default, Bun bundles all your code into a single .js
file, which can cause issues with lazy loading and accessing methods or variables from third-party packages due to the disrupted execution order. When bundling, we added the --splitting
command, which allows our files to be truly lazily loaded, especially useful when your project becomes very large.