Electron
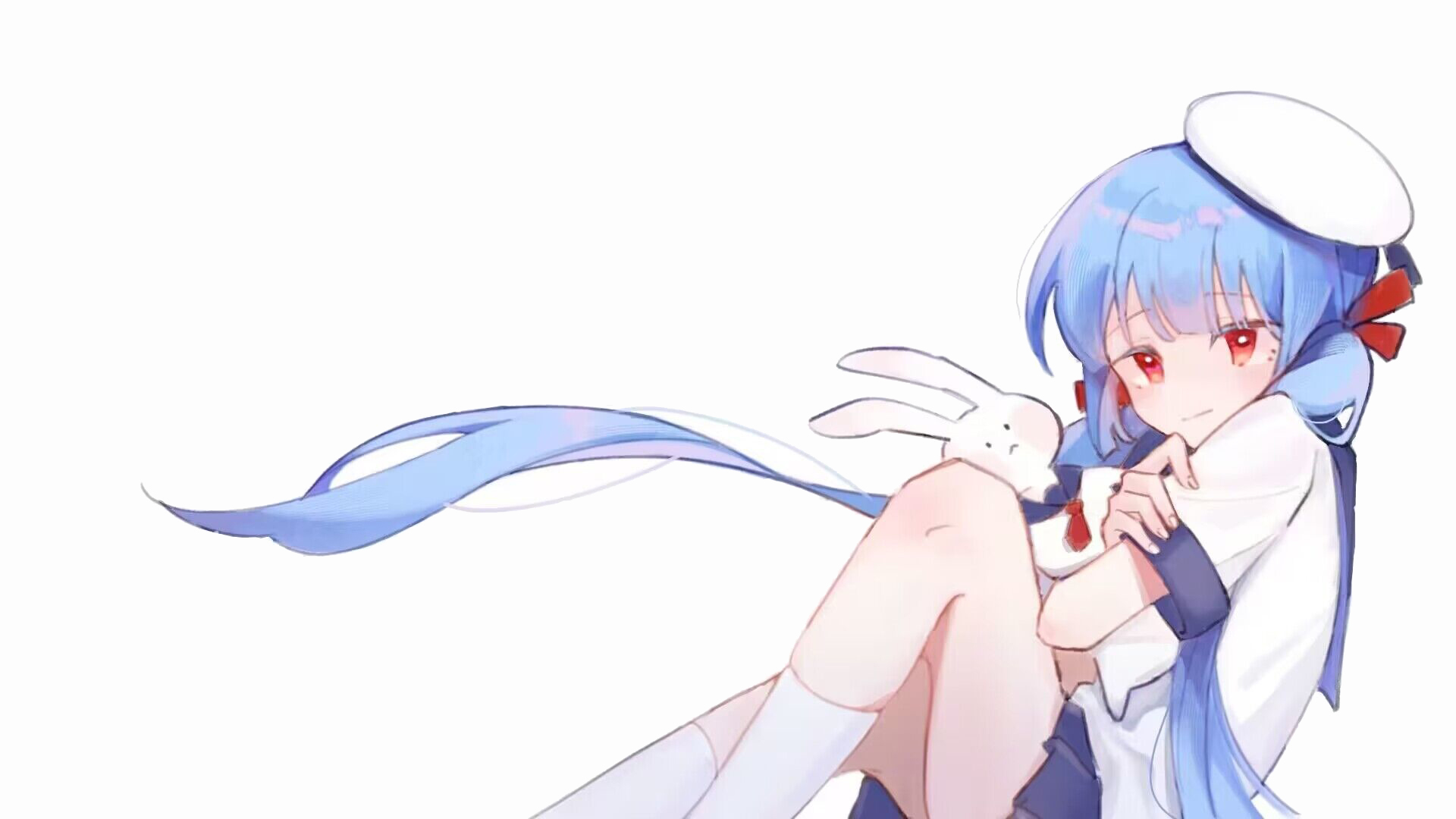
Milkio provides a solution for running in the Electron environment, empowering you to develop powerful desktop applications.
In traditional Electron application development, the communication mechanism between the main process and the renderer process has always been a key challenge. Common issues include a lack of type safety, the need to convert variables to string format, the requirement to write transitional code in the preload
script for data transfer, and the complexity of implementing bidirectional communication. These issues can potentially impact development efficiency and application performance.
With Milkio, you can start an HTTP server on a random available port, giving the renderer process the ability to call Electron or Node.js while ensuring security and enabling simple bidirectional communication.
Getting Started
When creating an application, choose electron
as the runtime environment.
bun create milkio
Runtime
Your code will run within Electron. For the main process, you are running in Node.js, and for the renderer process, you are running in the browser. Bun serves as a package manager and bundler during your Electron development. Therefore, ensure that your code can run in the environment it is in (Node.js or browser).
Page Development
After creating your application, by default, Astro is used as the page development framework. It is a Static Site Generator (SSG) framework that pre-renders the initial screen content of your page as HTML to speed up your page loading. In Astro, besides using the .astro
template to develop your pages, you can also use any framework such as Vue3, React, Preact, Svelte, Solid, Alpine, Lit, etc., to develop your pages.
The “drawback” of SSG frameworks is that not all code runs in the browser; some code may be pre-rendered. If you use browser-specific APIs in this code, issues may arise. If you find this cautious handling cumbersome for your complex application, you can ensure your components are always rendered in the browser by adding the client:only
directive in your .astro
file.
<!-- This component will always be rendered in the browser and not pre-rendered --><MyReactComponent client:only />
If you are developing a complex application and want to build a regular Single Page Application (SPA), you can treat Astro as a typical Vite application for development.
You can simply consider your /src/pages/index.astro
as index.html
and then include your main entry file by adding <script type="module" src="/src/main.ts"></script>
to it.
Within this setup, you can do anything. For example, if you are a Vue 3 developer, you can install Vue and use Vue Router in /src/main.ts
.
You can even mix both approaches, developing the main parts in an SPA manner and using Astro for features that are more display-oriented with light interactivity. You can think of Astro as a Vite with multiple page versions.
Electron
On the Electron official website or in some articles, we often see Electron functionality being used in the following way:
import { app } from "electron";app.whenReady();
However, in Milkio, you should rewrite it as follows:
electron.app.whenReady();
This is because we reduce the application size through bundling and enhance performance by compressing the code, bundling all dependencies together.
However, Electron is a “special” dependency. Importing it in the former way will result in Electron being bundled, leading to errors during distribution.
Assets
Electron Forge packages your source code into asar
format files, which efficiently run on systems with slow I/O (such as Windows).
Sometimes, we need to carry certain resources and want them to be stored in the file system as they are, rather than being packed into asar
. For example, icons, executable files, etc.
You can place these resources in the /assets
directory and in your code, you can obtain the absolute path of a resource using the following method:
import { join } from "node:path";import { configMilkio } from "/src/config/milkio";
const assetsPath = join(configMilkio.assets(), "your-asset.png");
Distribution
You can distribute your application by running the bun run build
command. The packaged installation package of your application will be stored in the /out
directory.
In this process, we utilize Electron Forge, which is the tool introduced by Electron for packaging and distribution.