Redis
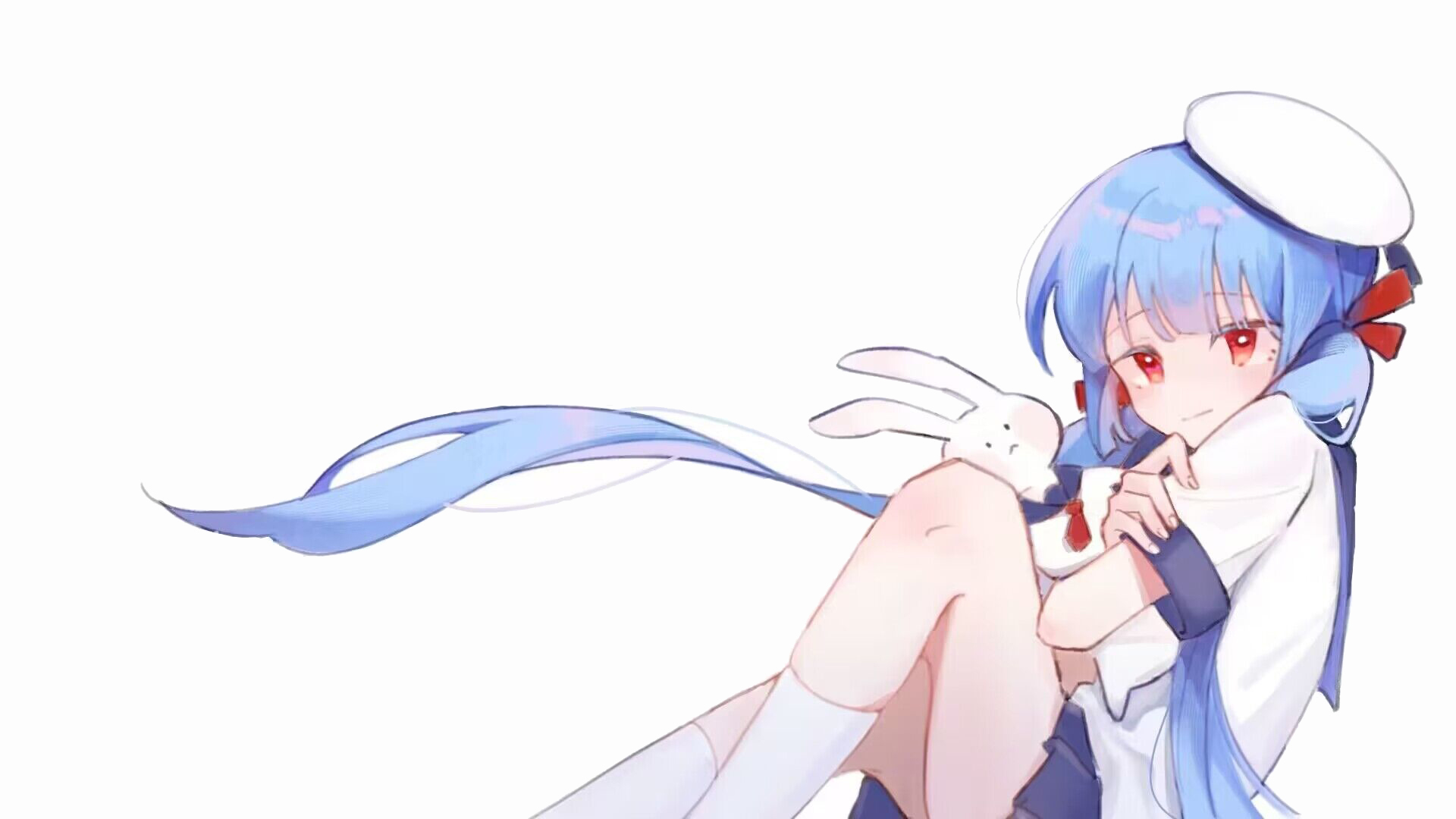
Redis is a battle-tested distributed caching database. It can temporarily cache certain data and automatically expire it when appropriate. By cleverly using it, you can significantly improve the performance of your application.
Getting Started
We need to install the package that combines Milkio with Redis:
npm i milkio-redis
In your /src/uses
directory, create a redis.ts
file and paste the following code into it. Don’t forget to modify the URL. If you’re not sure what URL to set, please ask your Redis service provider.
import { defineRedisClient } from "milkio-redis";import { configRedis } from "../config/redis";
export const redisClient = defineRedisClient({ url: "redis://127.0.0.1:6379", initTimeout: 3000,});
Location
Typically, we recommend creating a /src/caches
directory and placing your caches there. This also makes it convenient to share caches between multiple APIs.
Cache
You can use the defineCache
method to define a cache. For example, you can cache a boolean value to indicate whether the maintenance mode is enabled.
import { defineCache } from "milkio-redis";import { redisClient } from "../uses/redis-client";
const cache = defineCache<boolean>(redisClient, "enable-maintenance-mode");
await cache.set(true, 100);console.log(await cache.get());// output: true
Namespace Cache
Sometimes, we need to store collection data, such as creating caches for each user’s personal information. This allows for efficient data caching on a per-user basis.
import { defineNamespaceCache } from "milkio-redis";
const cache = defineNamespaceCache<UserInfo>(redisClient, "user-info");type UserInfo = { age: number; email: string;};
await cache.set("alice", { age: 18, email: "alice@gmail.com" }, 100);console.log(await cache.get("alice"));// output: { age: 18, email: 'alice@gmail.com' }
await cache.del();console.log(await cache.get());// output: undefined
Result Cache
Result caching allows you to cache the results of function executions. For example, you can cache the results of SQL queries or cache some time-consuming calculations to reduce duplicate work and improve response speed. In the code below, we pass 4 parameters: the key name, cache expiration time, actual expiration time of the cache, and a function as a parameter. The function will not be executed repeatedly until the cache expires, but the result will be obtained from the cache.
Since result caching is usually closely related to code logic, we generally do not put result caches in the /src/caches
directory.
const result = defineResultCache(redisClient, "your-key", 60, 60, async () => { const now = new Date().getTime(); await new Promise((resolve) => setTimeout(resolve, 1000)); // wait for 1 second return now;});console.log(await newYAML.getResult());
When the defineResultCache
code is executed, if the cache has not expired, the result will be retrieved from the cache. If the cache has expired, the method will be executed to get the result and re-cache it.
To reduce the probability of cache avalanche, you need to set two expiration times: TTL
and realTTL
.
When TTL
has not expired, it enters the normal cache stage, and all results come from the cache.
When TTL
has expired, it enters the cache expiration stage. During this stage, Milkio allows one request to recompute the result. Other requests still get the old cached data until the recomputation stage is finished and the cache is updated, returning to the normal cache stage.
Recomputation can fail, causing it to return to the cache expiration stage. The next arriving request will reenter the recomputation stage. By default, the recomputation stage lasts for 6 seconds. If it exceeds this time, it is considered a recomputation failure. You can modify the timeout time with options.realGetInterval
.
If there are no requests to get the result for a considerable period of time or continuous failures occur until the expiration time of TTL + realTTL
, the cached result will be completely deleted.
Getting the Native Redis Client
We can directly use the native Redis Client to operate Redis. You can use all Redis commands. Like this:
(await redisClient).HSET("key", "field", "value");
We recommend using the caching functionality carefully encapsulated by Milkio because it provides complete type support, making the code more efficient and simple to write, without having to worry about the type of values stored. Milkio will automatically serialize the values using TSON when storing and deserialize them when retrieving. With the native Redis client, you would need to handle these things manually.