Configuration
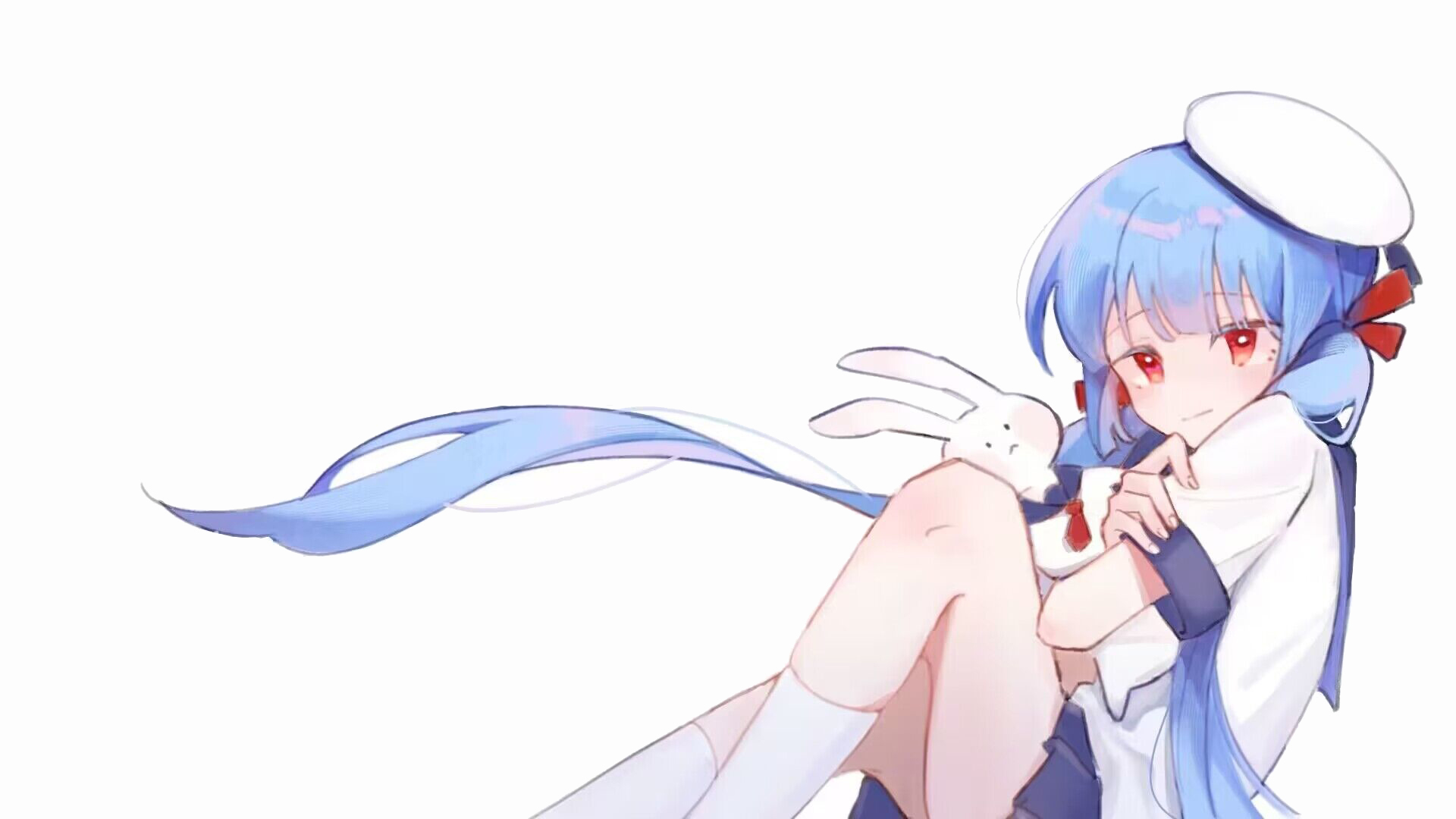
In development, it is common to need to manage various configuration information properly, such as database connection details, sensitive data like keys, and more.
Typically, we store this configuration information uniformly in the /src/config
directory of the project. Like this:
export const configExample = defineConfig(({ config }) => { return config({ foo: "bar", }).done();});
Based on this, we can easily import the configuration object wherever needed. It is a common practice that all configuration object names start with the prefix config
, so when you type config
in the code editor, you can quickly find all available configuration options.
import { configExample } from "/src/config/example";
console.log(configExample.foo); // output: "bar"
Environment
Perhaps in different environments, we need to use different values. For example, in a production environment (i.e., when running on a cloud server), we may need to use an intranet database connection address for faster speed and increased security. This can be easily achieved by specifying the environment.
export const configExample = defineConfig(({ config, mode }) => { return config({ foo: "bar", }) .environment(() => env.NODE_ENV === "development", { foo: "baz", }) .environment(() => env.NODE_ENV === "production", { foo: "qux", }) .done();});
The environment
method takes a method that returns a boolean as the first parameter. When the condition is met, the object in the second parameter will be shallow merged with your configuration.
In the example above, if you set export NODE_ENV=production
before running Milkio, then configExample.foo
will return "qux"
.
Edit Configuration
Configurations are not read-only; you can freely modify the configuration object. For example, in Cloudflare Workers or some other edge runtimes, they can only know the values in the environment variables when a request arrives. In this case, you can modify the values in your configuration object as needed in your /run-cloudflare.ts
or other runtime files.
import { configExample } from "/src/config/example";
configExample.foo = "qux";
Asynchronous Configuration
In some cases, you may need to fetch configuration information from a remote server, database, or Redis. Unlike text-based configurations like .env
, our configurations run directly in JavaScript, allowing you to set the configuration object as a Promise directly or pass in a self-executing method to fetch configuration information.
export const configExample = defineConfig(({ config }) => { return config({ foo: (async() => { return await fetch(...) })(), bar: "baz" }).done();});
Environment Variables
Environment variables are a common configuration method, but they lack type information, so the values we get from environment variables are all in string form. To help developers convert these strings into appropriate data types, the Milkio framework provides corresponding utility functions. These functions allow you to specify a default value to use if the environment variable is not defined.
import { env } from "node:process";import { envToBoolean, envToNumber, envToString } from "milkio";
export const configExample = { fooNumber: envToNumber(env.FOO, 9000), barBoolean: envToBoolean(env.BAR, false), bazString: envToString(env.BAZ, "hello-world"),};
.env Files
Some environments support using .env
files to automatically set environment variables, such as Bun.
Additionally, Bun supports using different .env
files by specifying a run mode, which is particularly useful during local development and deployment. By default, Bun reads the .env
file. To use a different set of environment variables during deployment, you can set the MODE
environment variable to the corresponding file name, e.g., .env.production
, or achieve the same effect by specifying --mode=.env.production
when running commands.
If you are using Node.js, the dotenv
package can help you achieve a similar effect.
Milkio Configuration File
When creating a new project, the Milkio framework automatically generates a configuration file named milkio.ts
, which contains configuration options related to the framework. You are free to modify these configurations as needed, such as adjusting default values.
Name | Environment Variable | Description |
---|---|---|
ignorePathLevel | MILKIO_IGNORE_PATH_LEVEL | Number of path levels to ignore, useful for addressing additional path issues generated during Serverless service deployment |
corsAllowMethods | MILKIO_CORS_ALLOW_METHODS | Sets the methods allowed for preflight requests by browsers, default is * |
corsAllowHeaders | MILKIO_CORS_ALLOW_HEADERS | Sets the headers allowed for preflight requests by browsers, default is * |
corsAllowOrigin | MILKIO_CORS_ALLOW_ORIGIN | Sets the origin allowed for preflight requests by browsers, default is * |
In this way, we can flexibly manage and configure our application to ensure its smooth operation in different environments.