Middleware
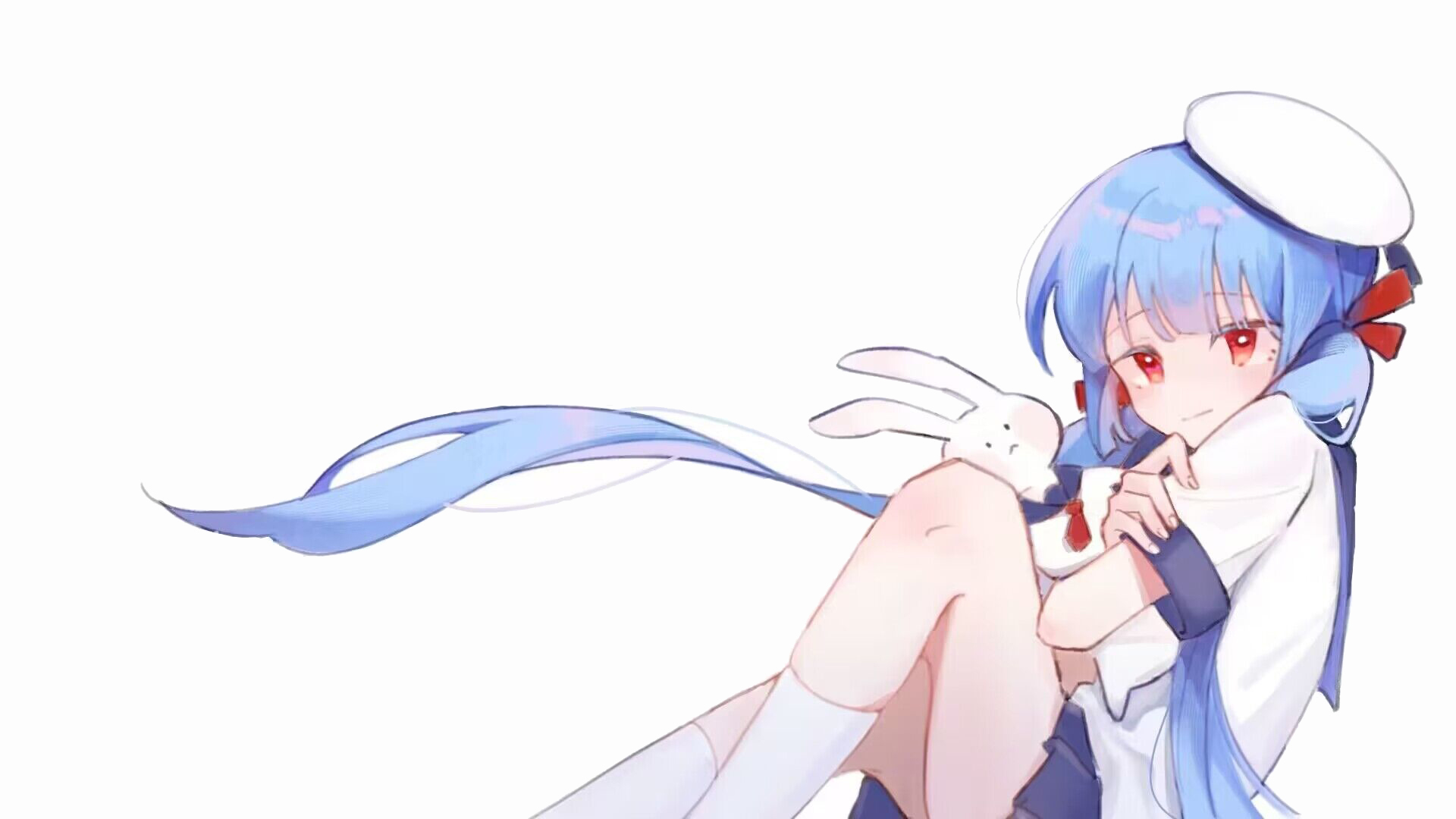
Middleware is a way to execute arbitrary logic globally before or after executing an API.
Typically, it is used in conjunction with Meta to inspect and filter requests and responses sent to the API, or to set up Context.
Initially, you wanted an API to only serve authenticated users, so you wrote code at the beginning of the API to check if the user is logged in:
export const api = defineApi({ meta: {}, async action(params: {}, context) { const token = context.headers.get("Authorization"); if (token !== "xxxx-xxxx-xxxx-xxxx") throw reject("DEVICE_NOT_LOGIN", "This device is not logged in yet"); // Your subsequent code.. },});
Later, you realized that this logic to ensure user authentication is needed for many APIs. In this case, you can refactor it into a middleware. Create a new file ensure-logined-middleware.ts
in your /src/middlewares/
directory:
export const ensureLoginedMiddleware = defineMiddleware({ beforeExecute: async (context) => { const token = context.headers.get("Authorization"); if (token !== "xxxx-xxxx-xxxx-xxxx") throw reject("DEVICE_NOT_LOGIN", "This device is not logged in yet"); },});
We use defineMiddleware
to define a middleware. Inside it, we use a beforeExecute
lifecycle hook, which is called before the API is executed. In any lifecycle hook, throwing an exception can interrupt the invocation. If all hook methods are executed successfully, the next hook will be executed.
We need to add the middleware to MilkioApp. Edit your /milkio.ts
file:
export const milkio = await createMilkioApp({ middlewares: () => [ // The middleware you just wrote.. ensureLoginedMiddleware(), ],});
middlewares
is a function that returns an array containing the middlewares you want to add to MilkioApp. The order of middlewares in the array determines their execution order, with the ones at the beginning being closer to the outer layer of the “onion.”
- middlewares: () => [middlewareA, middlewareB, middlewareC]
Client (Request)[ ▼ ](middlewareA -> beforeExecute)[ ▼ ](middlewareB -> beforeExecute)[ ▼ ](middlewareC -> beforeExecute)[ ▼ ]Execute API[ ▼ ](middlewareC -> afterExecute)[ ▼ ](middlewareB -> afterExecute)[ ▼ ](middlewareA -> afterExecute)[ ▼ ]Client (Response)
Lifecycle Hooks
Method Name | Trigger Point | Parameters | Remarks |
---|---|---|---|
bootstrap | Executed when MilkioApp starts | milkioApp | Executed only once at startup |
afterHTTPRequest | Executed after the HTTP request arrives | headers, detail | Executed only for HTTP calls |
beforeExecute | Executed before the API is actually called | context | Executed for any type of invocation |
afterExecute | Executed after the API is actually called | context, response | Executed for any type of invocation |
beforeHTTPResponse | Executed before the HTTP request ends | response, detail | Executed only for HTTP calls |